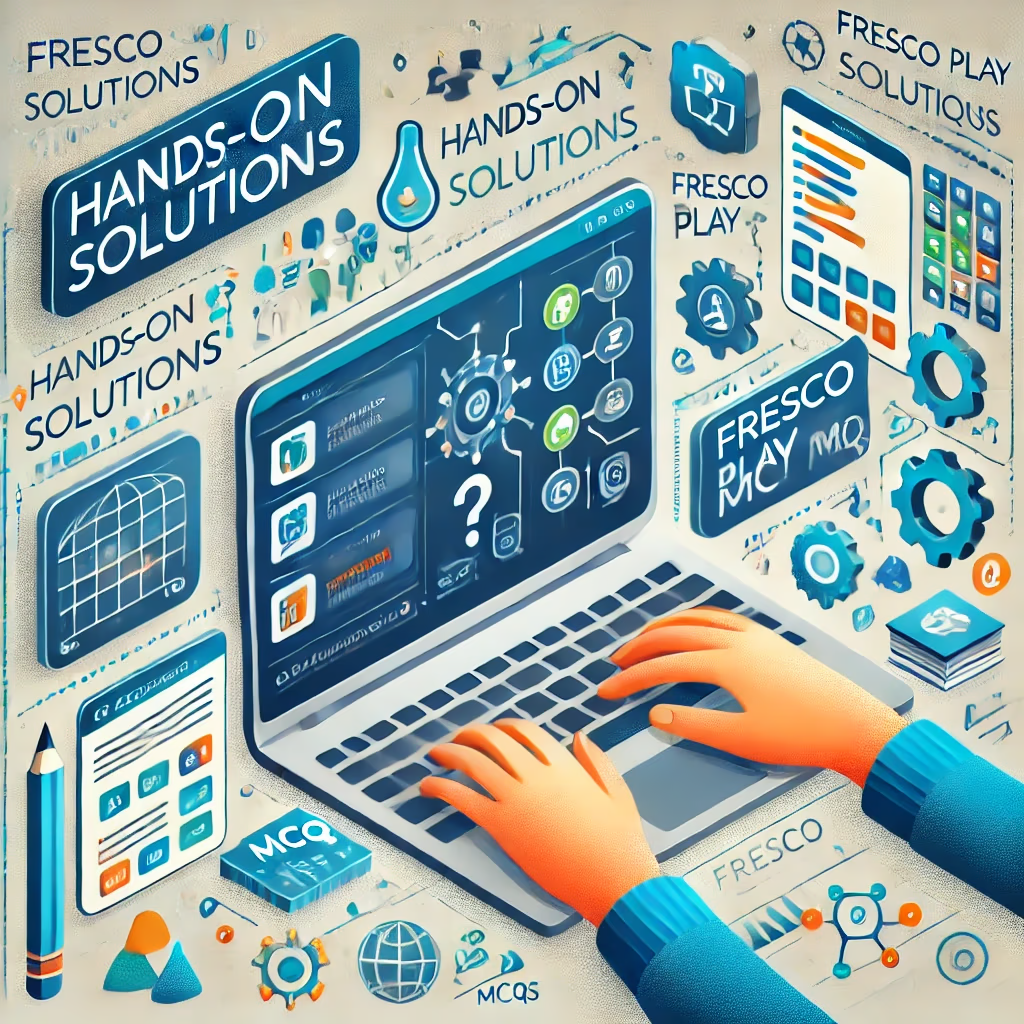
Dependency Injection Hands On Answer:
This hands- on is about providing your angular component with the required data, through A service. This challenge does not make any HTTP calls to take calls to get data.
Expected output
Contact applications
Rajesh
Oorjit
Anjjan
David
Answer:
contact-list.component.ts
ngOnInit() {
this.contactService.getContacts().subscribe(contactlst => {
this.contacts = contactlst.contactList;
}) ;
}
appmodule.ts
important { ContactService } from ‘./services/contact.service’;
providers: [ContactService],
contact.service.ts
getContacts(): Observable <Contacts> {
return of(this.contacts as Contacts) ;
}
Routers Hands on Answer:
This hands-on is about using routers in angular to
- redirect the user to a specific URL
- load necessary components based on a link clicked by the user
Expected Output:
Default home page should return home page.
Routing to /contacts should return contact list.
Routing to /cites should return cities list
Answer:
app-routing.module.ts
export const routes: Routes = [
{ path: ‘contacts’, component:ContactsComponent },
{ path: ‘cities’, component:CityComponent }];
@NgModule({
imports: [RouterModule.forRoots(routes)],
exports: [RouterModule]
})
contacts.component.ts, city.component.ts
constructor(public contactService:ContactService)
ngOnInit() {
this.contactService.getContacts().subscribe(contactlst => {
this.contacts = contactlst.contactList;
}) ;
}
contact.service.ts
@Injectable({
providedIn:’root’
})
url = ‘https://www.sample.in/12345’;
constructor(public http:HttpClient) {}
getContacts() : Observable<Contacts> {
return this.http.get<Contacts>(this.url);
}
Angular Reactive Forms Hands On Answer:
Forms are the most popular way to accept value from a user.
This hands-on is about creating a form using Reactive Forms. You also need to add necessary validations to each of the form fields
The details of the validation to be added are given in app.component.ts file.
contactForm = new FormGroup ({
name:new FormControl( ‘ ‘ , [ Validators.required]),
phone: new FormControl( ‘ ‘ , [ Validators.required, Validators.pattern(“^[0-9]*$), Validator.minLength(10), Validator.maxLength(10)]),
address: new FormGroup({
street: new FormControl(),
city: new FormControl(),
zip:new FormControl(‘ ‘, Validators.pattern(“^[0-9]*$), Validator.minLength(6), Validator.maxLength(6)])
})
Disclaimer: The main motive to provide this solution is to help and support those who are unable to do these courses due to facing some issue and having a little bit of a lack of knowledge. All of the material and information contained on this website is for knowledge and education purposes only.