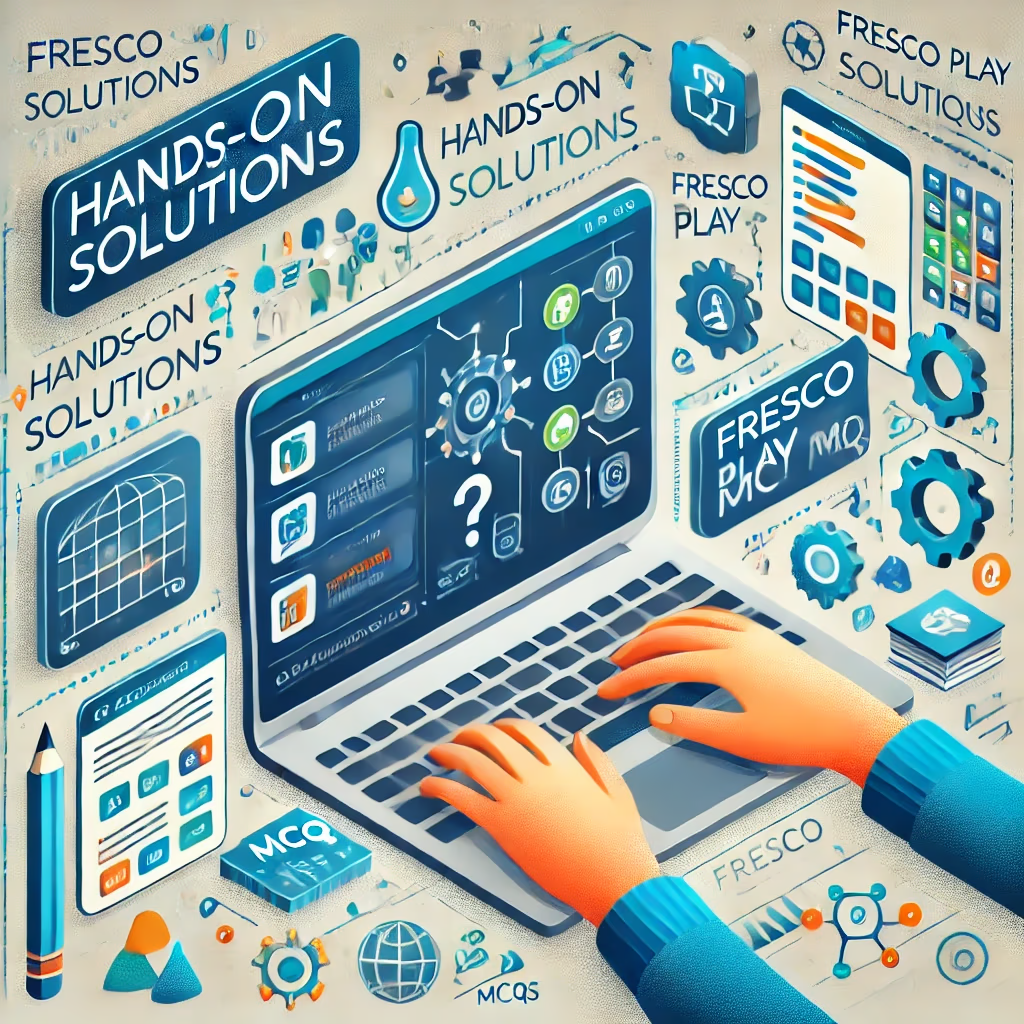
jQuery:
JQuery is a popular JS library developed by John Resig.
JQuery is a JavaScript library. It is a free, highly featured open source software designed to make client- side programming of HTML simple with easy-to-use API.
The generic principle of jQuery is “Find something and do something to it using pre-written JavaScript”
jQuery Hands-on 1 Addition Answer:
Write a function named add() that accepts 2 numbers from users, and returns the sum (integer) of the same.
Get the values of the input fields (IDs #num1 and #num2) from the user, and display the sum in the input field ID #total when the button is clicked.
For invalid inputs, lthe result should be displayed as ‘NaN’.
Answer:
function add() {
var a = $(“#num1”).val();
var b = $(“#num2”).val();
if(isNaN(a) || isNaN(b)
{
$(“#total”).val(‘NaN’) ;
} else {
var c = parseInt(a) + parseInt(b);
$(“#total”).val(c) ;
}
};
jQuery Hands-on 2 Login Page Answer:
Write a function login() that will read the username and password from the text boxes IDs #username, #password, and validate them.
For username = “admin” and password=”password” create an alert box “You are valid user” or “You are not a valid user”.
Answer:
function login()
{
var username = $(‘#username’).val();
var password = $(‘#password’).val();
if(username === ‘admin’ && password === ‘password’) {
alert(“You are valid user) ;
} else {
alert(“You are not a valid user”) ;
}
};
jQuery Hands-on 3 Dom Manipulation – Home Page Answer:
Write a function login() that will redirect to home.html after clicking the login button.
Use username=”admin”, and password=” password”. Else, show an alert message “You are not a valid user”.
Make user you use the doRedirect() function provided in the index.js file to redirect from index.html to home.html.
Answer:
function login()
{
var username = $(‘#username’).val();
var password = $(‘#password’).val();
if(username === ‘admin’ && password === ‘password’) {
doRedirect(‘home.html’) ;
alert(“You are valid user) ;
} else {
alert(“You are not a valid user”) ;
}
};
function doRedirect(href) {
window.location = href;
}
jQuery Hands-on 4 Animation Answer
function hover1()
{
$(“#item1-des”).slideToggle() ;
}
function hover2()
{
$(“#item2-des”).slideToggle() ;
}
function animate_big()
{
$(“#buy”).animate({width:”200px”}, 500)
}
function animate_small()
{
$(“#buy”).animate({width:”100px”}, 500)
}
jQuery Hands-on 5 AJAX
Write a function load_des() which can load content for the div tag #item1-des from the URL:
“http://xxxxxxx/yyyyyy/zzz.html”.
function load_des()
{
$(“#item1-des”).load(“http://xxxxxxx/yyyyyy/zzz.html”);
}
Disclaimer: The main motive to provide this solution is to help and support those who are unable to do these courses due to facing some issue and having a little bit lack of knowledge. All of the material and information contained on this website is for knowledge and education purposes only.